Getting Started
Let's integrate the UseCSV Importer plugin with your Vue.js app.
Make sure you configured your importer before starting.
Install
Using NPM
Vue 2
npm install @usecsv/vuejs
Vue 3
npm install @usecsv/vuejs3
Using YARN
Vue 2
yarn add @usecsv/vuejs
Vue 3
yarn add @usecsv/vuejs3
Add the Importer
Adding the UseCSVButton
component to your frontend will render a button that triggers the import modal when clicked.
Code example with default button
Check codesandbox example.
Vue 2
// main.js
import UseCSVButton from "@usecsv/vuejs";
Vue.use(UseCSVButton);
// App.vue
<template>
<usecsv-button importerKey="your-importer-key">Import Data</usecsv-button>
</template>
Vue 3
// global registration
// main.js
import { createApp } from "vue"
import App from "./App.vue"
import UseCSVButton from "@usecsv/vuejs3"
createApp(App).component("usecsv-button", UseCSVButton).mount("#app")
// App.vue
<template>
<usecsv-button importerKey="your-importer-key">
Import Data
</usecsv-button>
</template>
// local registration
// App.vue
<script>
import UseCSVButton from "@usecsv/vuejs3"
export default {
components: {
"usecsv-button": UseCSVButton
}
}
</script>
<template>
<usecsv-button importerKey="your-importer-key">
Import Data
</usecsv-button>
</template>
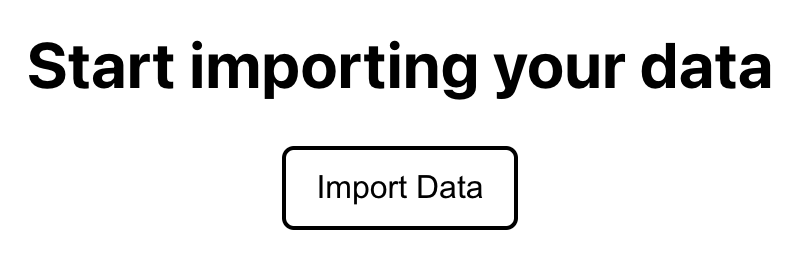
Code example with custom button
Use scoped slots to render your custom button component.
The component has to have an openModal
prop which is passed down from usecsv-button
Vue 2
Check codesandbox example.
// main.js
import UseCSVButton from "@usecsv/vuejs";
Vue.use(UseCSVButton);
// App.vue
<template>
<usecsv-button importerKey="your-importer-key" v-slot="slotProps">
<button class="btn" @click="slotProps.openModal">Import Data</button>
</usecsv-button>
</template>
<script>
export default {
name: "App",
};
</script>
<style>
.btn {
width: 170px;
height: 70px;
color: #fff;
background-color: #027ad6;
box-shadow: 0 32px 64px rgba(0, 0, 0, 0.07);
font: 700 24px sans-serif;
text-align: center;
border: none;
border-radius: 3px;
}
</style>
Vue 3
Check codesandbox example.
// global registration
// main.js
import { createApp } from "vue"
import App from "./App.vue"
import UseCSVButton from "@usecsv/vuejs3"
createApp(App).component("usecsv-button", UseCSVButton).mount("#app")
// App.vue
<template>
<usecsv-button importerKey="your-importer-key" v-slot="slotProps">
<button class="btn" @click="slotProps.openModal()">Import Data</button>
</usecsv-button>
</template>
<style>
.btn {
/* ...your css styles */
}
</style>
Check codesandbox example.
// local registration
// App.vue
<script>
import UseCSVButton from "@usecsv/vuejs3"
export default {
components: {
"usecsv-button": UseCSVButton
}
}
</script>
<template>
<usecsv-button importerKey="your-importer-key" v-slot="slotProps">
<button class="btn" @click="slotProps.openModal()">Import Data</button>
</usecsv-button>
</template>
<style>
.btn {
/* ...your css styles */
}
</style>
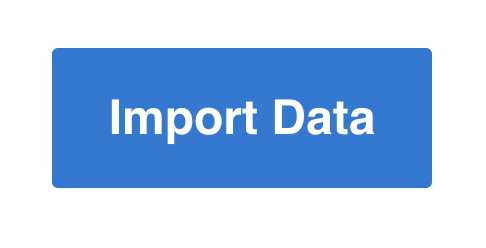
Now your users can import data from within your app, the final step is to setup a webhook in your backend to receive imports. Let's goto the Webhook docs.