Getting Started
Let's integrate the UseCSV Importer plugin with your app.
Make sure you configured your importer before starting.
Using UseCSV
useCsvPlugin
can be used as a function to trigger the import modal when called.
1. Install
Using NPM
npm install @usecsv/js
Using YARN
yarn add @usecsv/js
Code example
Check codesandbox example.
index.html
<html>
<head>
<title>UseCSV</title>
<meta charset="UTF-8" />
</head>
<body>
<div>
<h1>Start importing your data</h1>
<button type="button" id="import-data-button" onclick="importData()">
Import Data
</button>
</div>
<script src="index.js"></script>
</body>
</html>
index.js
import useCsvPlugin from "@usecsv/js";
function importData() {
useCsvPlugin({
importerKey: "your-importer-key",
user: { userId: "12345" },
metadata: { anotherId: "1" },
});
}
2. Reference the CDN
<script src="https://unpkg.com/@usecsv/js/build/index.umd.js"></script>
Code example
Check codesandbox example.
index.html
<html>
<head>
<title>UseCSV</title>
<meta charset="UTF-8" />
</head>
<body>
<div>
<h1>Start importing your data</h1>
<button type="button" id="import-data-button">Import Data</button>
</div>
<script src="https://unpkg.com/@usecsv/js/build/index.umd.js"></script>
<script type="text/javascript">
var importButton = document.getElementById("import-data-button");
importButton.onclick = function () {
useCsvPlugin({
importerKey: "your-importer-key",
user: { userId: "12345" },
metadata: { anotherId: "1" },
});
};
</script>
</body>
</html>
Code example ui is shown below
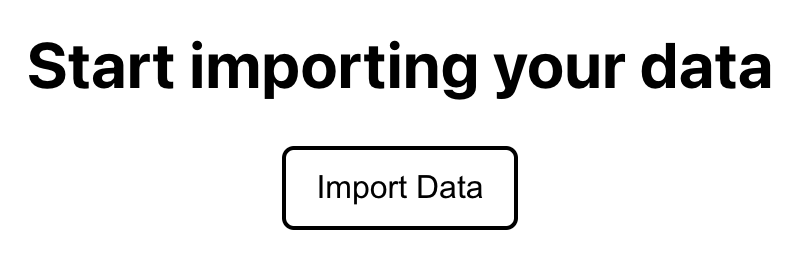
Now your users can import data from within your app, the final step is to setup a webhook in your backend to receive imports. Let's goto the Webhook docs.