Getting Started
Let's integrate the UseCSV Importer plugin with your React app.
Make sure you configured your importer before starting.
Install
Using NPM
npm install @usecsv/react
Using YARN
yarn add @usecsv/react
Add the Importer
Adding the UseCSV
component to your frontend will render a button that triggers the import modal when clicked.
Code example with default button
Check codesandbox example.
import UseCSV from "@usecsv/react";
const App = () => {
return (
<div>
<h1>Start importing your data</h1>
<UseCSV importerKey="your-importer-key" user={{ userId: "12345" }}>
Import Data
</UseCSV>
</div>
);
};
export default App;
The component contents will be used as the button text.
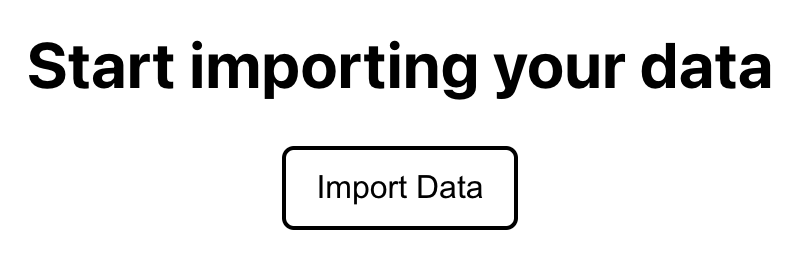
Code example with custom render prop
Check codesandbox example.
More details can be found in render prop
import UseCSV from "@usecsv/react";
function App() {
const renderButton = (openModal) => {
return (
<button
onClick={openModal}
style={{
width: "170px",
height: "70px",
color: "#fff",
backgroundColor: "#027ad6",
boxShadow: "0 32px 64px rgba(0,0,0,0.07);",
font: "700 24px sans-serif",
textAlign: "center",
border: "none",
borderRadius: "3px",
}}
>
Import Data
</button>
);
};
return (
<div className="App">
<UseCSV
importerKey="your-importer-key"
user={{ userId: 12345 }}
render={(openModal) => renderButton(openModal)}
/>
</div>
);
}
export default App;
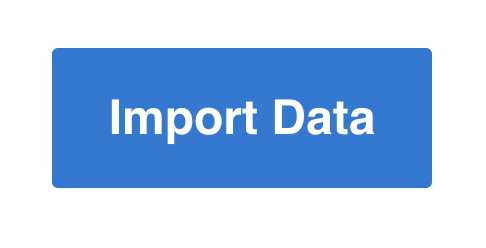
Now your users can import data from within your app, the final step is to setup a webhook in your backend to receive imports. Let's goto the Webhook docs.